Django Class Based Views
From Django 1.3, The concept of class based views has come up. In this way, view functions are replaced by Python objects.
Specific HTTP methods can be determined by separate methods and can be used more like object-based techniques (mixins).
Class Based Views
First, let’s see the difference between the function and method in Python with an example.:
1 2 3 4 5 | class PrintMe(object): def __init__(self, astring): self.l = astring def print_me(self): print(self.l) |
Let’s run the method:
1 2 | p = PrintMe("po") p.print_me() |
Function
1 2 | def print_me(l): print l |
Execute function:
1 | print_me("po") |
Output;
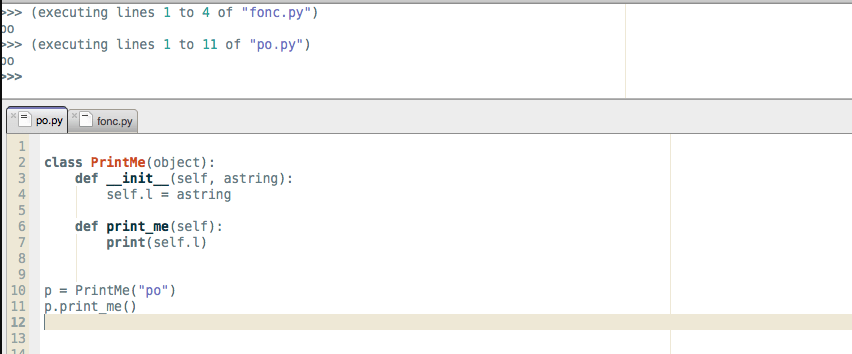
For an HTTP GET on Django , a view function looks like this:
1 2 3 4 5 6 | from django.http import HttpResponse def any_view(request): if request.method == 'GET': # kodlarınız return HttpResponse('any responce') |
While in CBV (class-based views) :
1 2 3 4 5 | from django.views.generic import View class AnyView(View): def get(self, request): # kodlarınız return HttpResponse('any responce') |
Urls.PY
1 2 3 4 5 6 | from django.conf.urls import patterns from anyapp.views import AnyView urlpatterns = patterns('', (r'^any/', AnyView.as_view()), ) |
Get and post methods in CBV:
On a CBV, the arguments of the Get and POST method are self, request (HttpRequest), and the request argument you specify in Urlconfig.:
1 2 3 4 5 6 | from django.views.generic import View class ViewClass(View): def get(self, request, arg1, keyword=value): return method1() def post(self, request, arg1, keyword=value): return method2() |
Source;
https://docs.djangoproject.com/en/dev/topics/class-based-views/intro/
http://lgiordani.github.io/blog/2013/10/28/digging-up-django-class-based-views-1/
http://programmer.97things.oreilly.com/wiki/index.php/Don’t_Repeat_Yourself